Scientific notation isn't helpful when you are trying to make quick comparisons across your DataFrame, and when your values are not that long. However, Pandas will introduce scientific notation by default when the data type is a float. Here is a way of removing it.
What is Scientific Notation?
Scientific notation (numbers with e) is a way of writing very large or very small numbers. A number is written in scientific notation when a number between 1 and 10 is multiplied by a power of 10.
For example:
- 2.3e-5, means 2.3 times ten to the minus five power, or 0.000023
- 4.5e6 means 4.5 times ten to the sixth power, or 4500000 which is the same as 4,500,000
This is a notation standard used by many computer programs including Python Pandas. This is simply a shortcut for entering very large values, or tiny fractions, without using logarithms.
How Scientific Notation Looks in Pandas
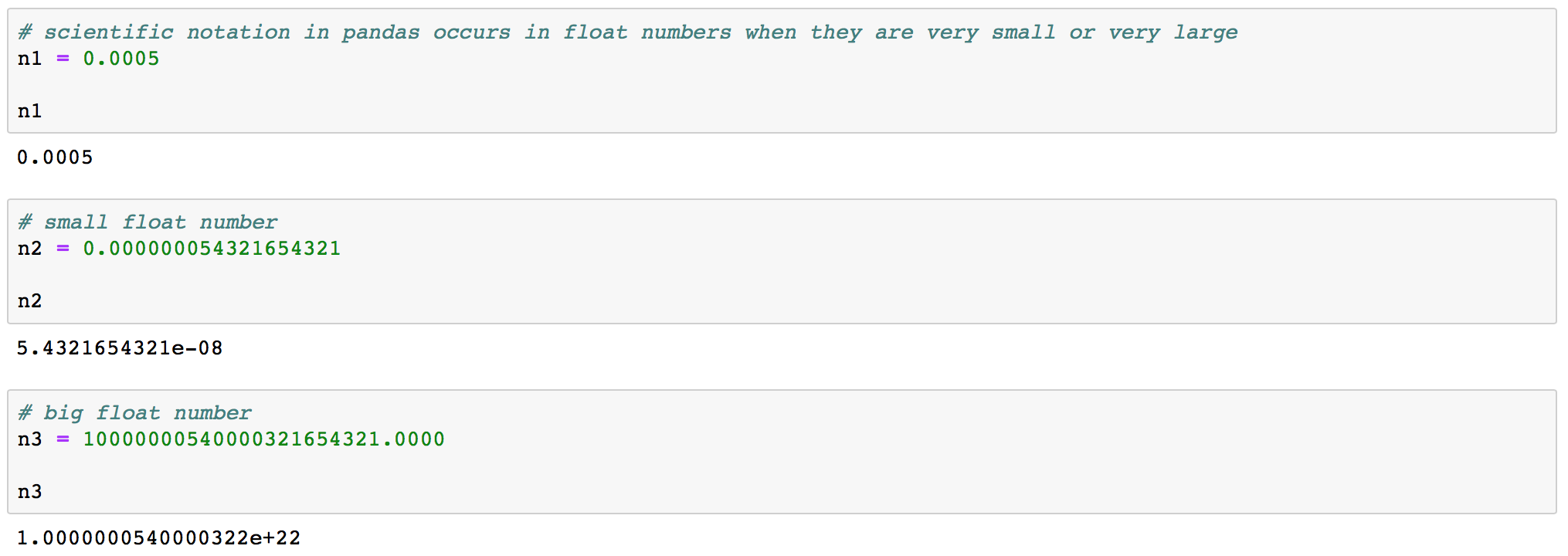
Let's create a test DataFrame with random numbers in a float format in order to illustrate scientific notation.
df = pd.DataFrame(np.random.random(5)**10, columns=['random'])
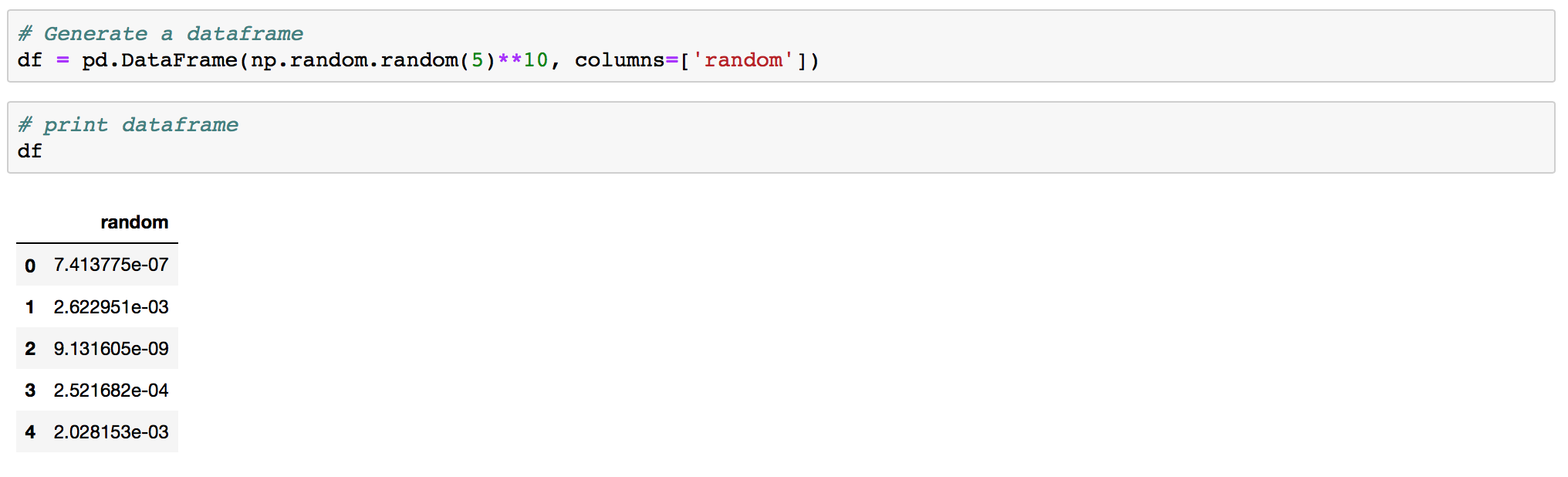
As we can see the random column now contains numbers in scientific notation like 7.413775e-07. If you run the same command it will generate different numbers for you, but they will all be in the scientific notation format. This happens since we are using np.random
to generate random numbers.
How to suppress scientific notation in Pandas
There are four ways of showing all of the decimals when using Python Pandas instead of scientific notation.
Solution 1: use .round()
df.round(5)
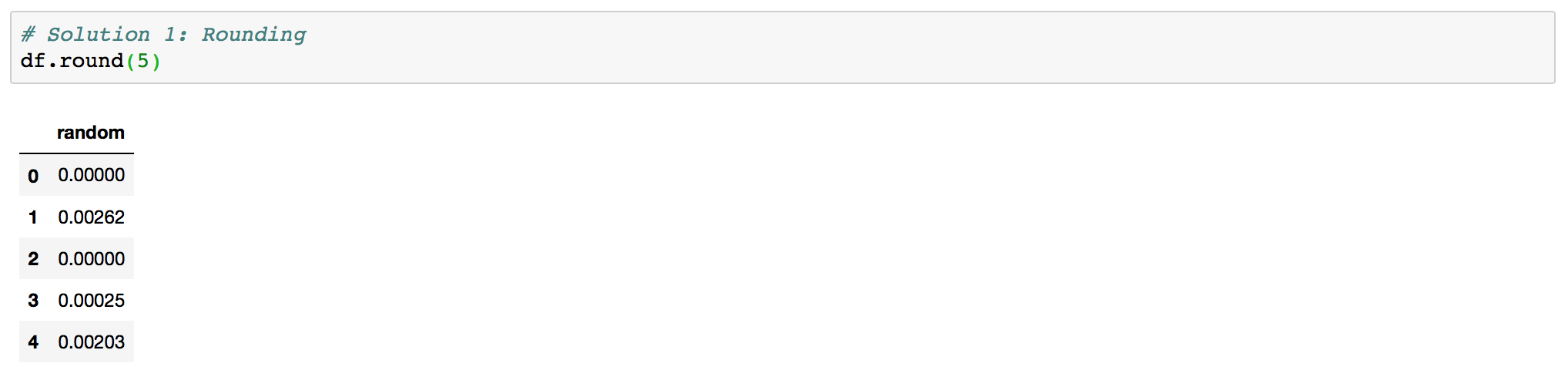
Solution 2: Use apply to change format
df.apply(lambda x: '%.5f' % x, axis=1)

Solution 3: Use .set_option()
Note that .set_option()
changes behavior globaly in Jupyter Notebooks, so it is not a temporary fix.
pd.set_option('display.float_format', lambda x: '%.5f' % x)

In order to revert Pandas behaviour to defaul use .reset_option()
.
pd.reset_option('display.float_format')
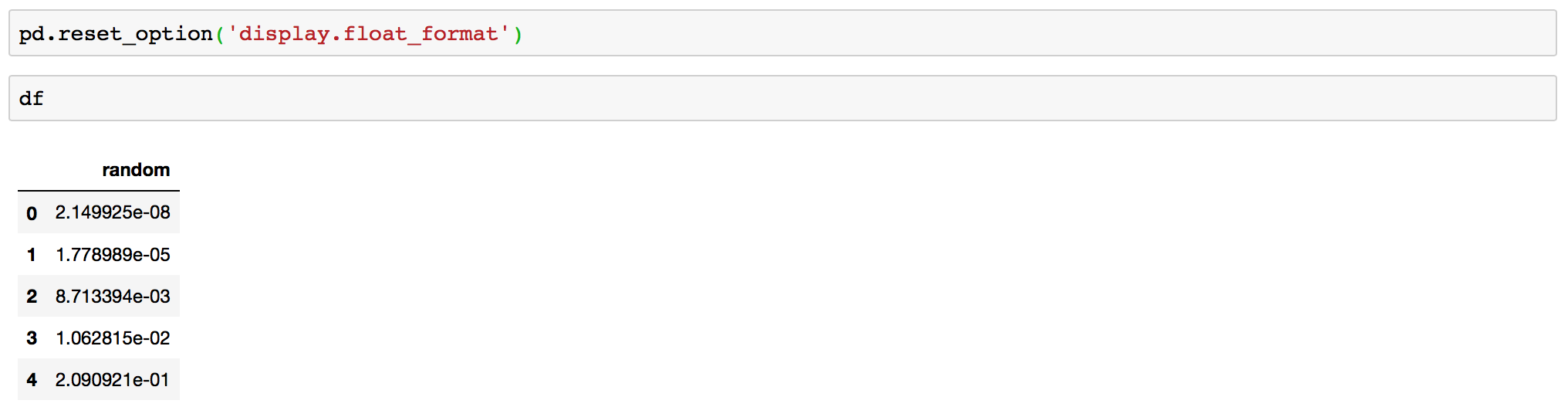
Note that the DataFrame was generated again using the random command, so we now have different numbers in it.
Solution 4: Assign display.float_format
You can change the display format using any Python formatter:
pd.options.display.float_format = '{:.5f}'.format
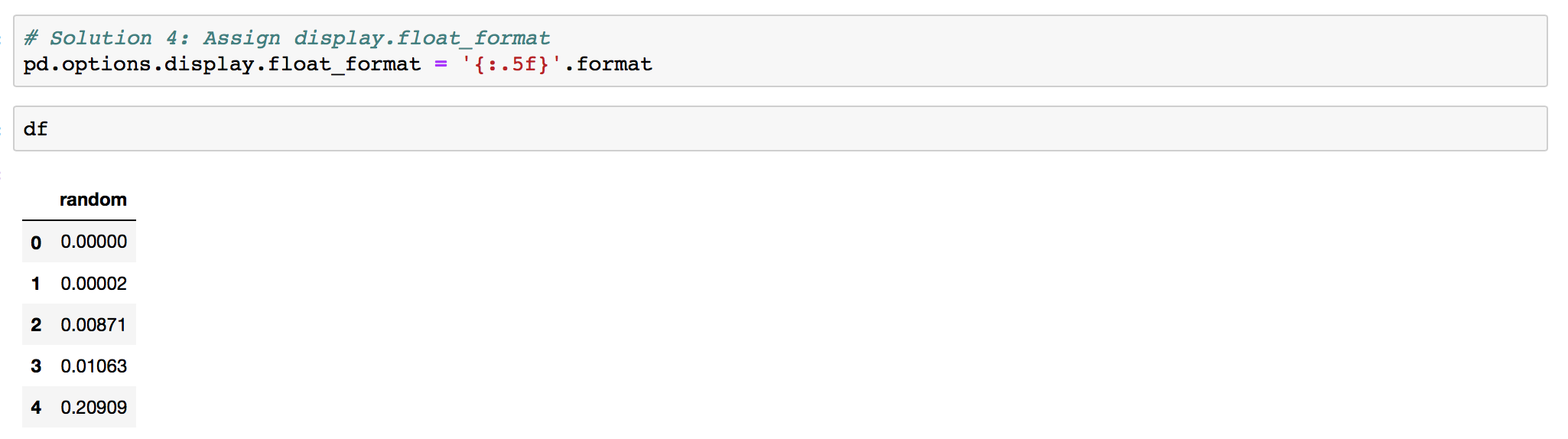
To revert back, you can use pd.reset_option
with a regex to reset more than one simultaneously. In this case to reset all options starting with display
you can:
pd.reset_option('^display.', silent=True)
Now that you know how to modify the default Pandas output and how to suppress scientific notation, you are more empowered.