Accessing a single value or updating the value of single row is sometime needed in Python Pandas Dataframe when we don't want to create a new Dataframe for just updating that single cell value. The easiest way to to access a single cell values is via Pandas in-built functions at
and iat
.
Pandas loc vs. iloc vs. at vs. iat?
If you are new to Python then you can be a bit confused by the cell localization/selection in Pandas. Don't worry if you are struggling to understand the practical implications of the various localization/selection options. Here is a quick explainer of why you should ever use .loc
or .iloc
over at
, and iat
or vice versa? And in what situations should you use which method?
loc: only work on index
iloc: work on position
at: get scalar values. It's a very fast loc
iat: Get scalar values. It's a very fast iloc
at
and iat
are meant to access a scalar, that is, a single element in the dataframe, while loc
and iloc
are ments to access several elements at the same time, potentially to perform vectorized operations.
Forget about .ix!
Please note that before pandas v0.20 a function called .ix
used to exist. This method was later split into two loc
and iloc
to make the explicit distinction between positional and label based indexing. Please beware that .ix
was discontinued due to inconsistent behavior and being hard to grok, and no longer exists in current versions of pandas (>= 1.0). So you can ignore this one.
How Pandas makes selections from a DataFrame
There are two primary ways that Pandas makes selections from a DataFrame.
By Label By Integer Location
There are three primary indexers for pandas. We have the indexing operator itself (the brackets []
), .loc
, and .iloc
. Let’s summarize them:
[]
- Primarily selects subsets of columns, but can select rows as well. Cannot simultaneously select rows and columns.
.loc
- selects subsets of rows and columns by label only
.iloc
- selects subsets of rows and columns by integer location only
.at
selects a single scalar value in the DataFrame by label only
.iat
selects a single scalar value in the DataFrame by integer location only
It is worth noting that .at
and .iat
are very similar to .loc
, and .iloc
. They have same functionality with just a small performance increase. Use those when you have a very time-sensitive application.
In addition to selection by label and integer location, boolean selection also known as boolean indexing exists.
Update Pandas Dataframe cell value by Column Label
.at
- Access a single value for a row/column label pair Use at if you only need to get or set a single value in a DataFrame or Series.
We begin by creating a sample Dataframe:
# creating a simple dataframe
df = pd.DataFrame({'name': ['Olivia','Dean','Alex','Jon','Tom','Jane','Kate'],
'age': [32,23,45,35,20,28,55],
'sex':['female', 'male','male','male','male','female','female']})
This is how your test Dataframe will look like:
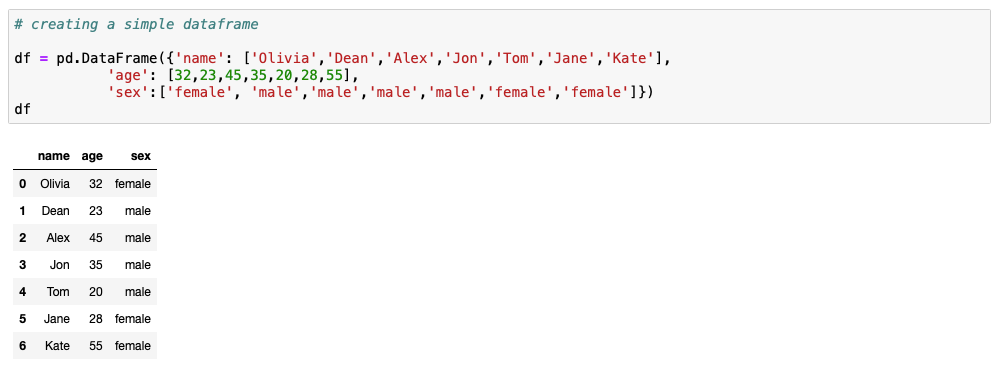
Let’s access cell value with index 2 and Column age. This is an age entry for Alex that is located at index 2.
# Let’s access cell value with index 2 and column age
df.at[2,'age']

Value 45 is the output when you execute the above line of code.
Now let’s update this value with 40.
# Now let's update cell value with index 2 and Column age
# We will replace value of 45 with 40
df.at[2,'age']=40
df
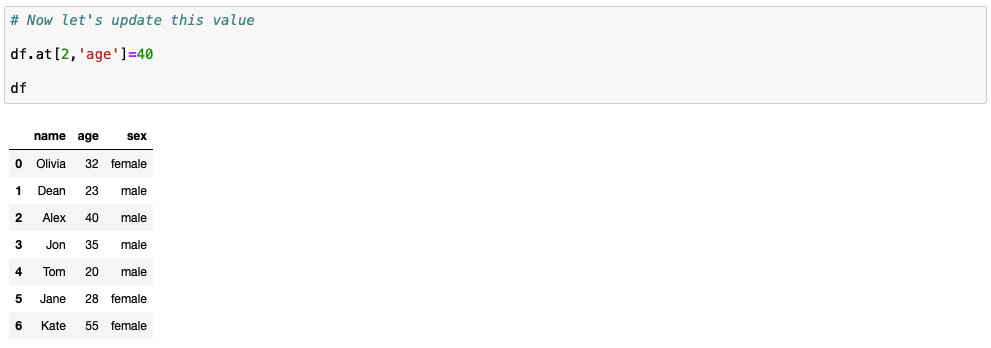
Now if you run the same comand we run to access cell value with index 2 and column age you will get 40 and not 45 that we had at the start.

You can access cell value via .loc
but you can't updated it this way!
df.loc[index].at['column']
or df.loc[index].at['column']

.loc
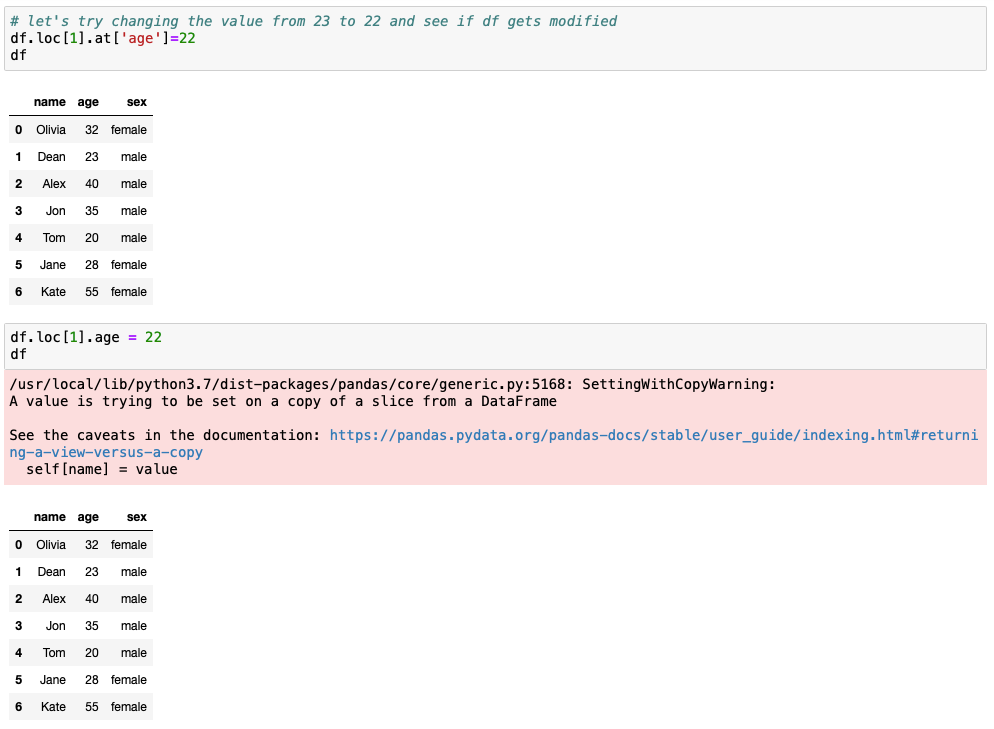
Dataframe cell value by Integer position
We can also do the same but instead of using column label we can use column index. In earlier exsample we used column label age which is the same as saying column 1. Don't forget that Python indexing starts at 0. For this we will use iat
.
iat
- Access a single value for a row/column pair by integer position. Use iat if you only need to get or set a single value in a DataFrame or Series.
# Let’s access cell value with index 1 and Column age (column index 1)
df.iat[1, 1]
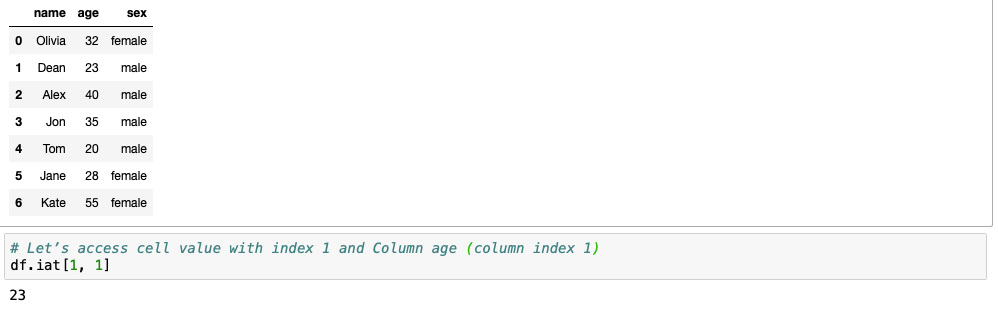
Now let's update cell value with index 1 and column index 1 to 22.
# Now let's update this value
df.iat[1, 1]=22
df
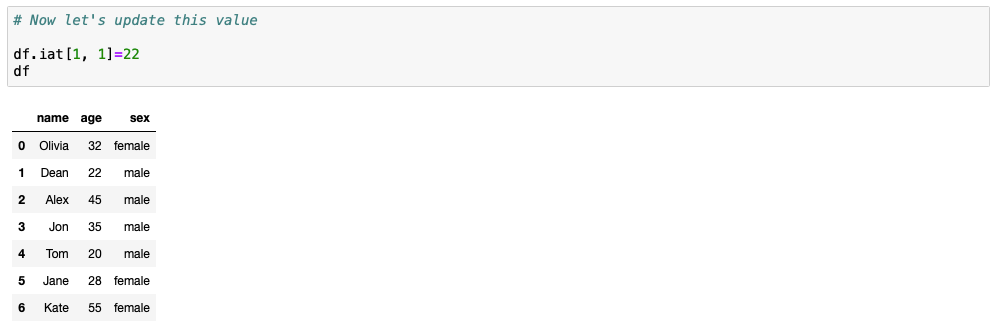
Conclusion
So you have seen how you can access a cell value and update it using at
and iat
which is meant to access a scalar, that is, a single element in the dataframe, while loc
and iloc
are meant to access several elements at the same time, potentially to perform vectorized operations. Advantage over loc is that this is faster and allows you to update cell value in your Dataframe.